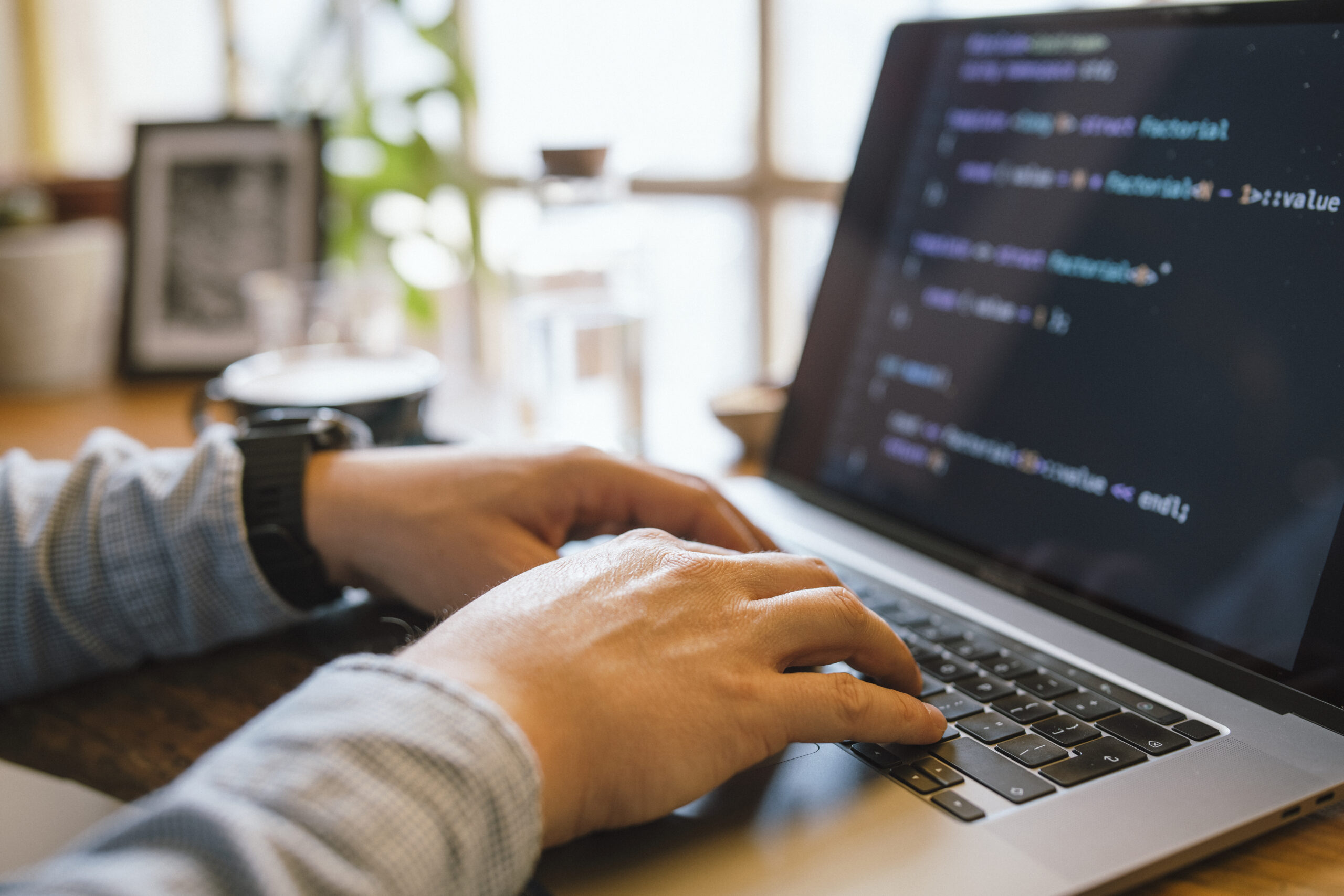
Debugging is Just about the most essential — but generally missed — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Improper, and Finding out to Assume methodically to unravel complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can save hours of frustration and significantly enhance your productivity. Here are several strategies that will help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the resources they use every day. While crafting code is one particular Portion of improvement, understanding ways to connect with it efficiently throughout execution is equally vital. Present day growth environments arrive equipped with impressive debugging abilities — but a lot of developers only scratch the area of what these instruments can do.
Consider, as an example, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, as well as modify code over the fly. When applied appropriately, they let you notice specifically how your code behaves during execution, that's priceless for monitoring down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-conclusion developers. They assist you to inspect the DOM, check community requests, look at serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over managing procedures and memory administration. Understanding these applications may have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Edition Management devices like Git to understand code background, locate the precise minute bugs were being released, and isolate problematic changes.
In the end, mastering your equipment signifies likely outside of default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The greater you are aware of your applications, the greater time you could expend resolving the particular problem instead of fumbling via the process.
Reproduce the Problem
Probably the most crucial — and often overlooked — steps in helpful debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers need to produce a regular setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a game of probability, typically resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as feasible. Check with queries like: What actions led to The difficulty? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it will become to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected plenty of details, seek to recreate the challenge in your local setting. This could signify inputting exactly the same facts, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the edge circumstances or point out transitions involved. These checks not just enable expose the problem but in addition reduce regressions Later on.
From time to time, The difficulty could be ecosystem-particular — it would materialize only on particular running units, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes securely, and communicate much more Obviously along with your group or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Read through and Recognize the Error Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should study to deal with error messages as direct communications within the process. They generally inform you just what occurred, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Commence by looking through the message carefully As well as in entire. Numerous builders, particularly when below time pressure, look at the initial line and immediately get started generating assumptions. But deeper from the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and realize them first.
Split the mistake down into areas. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a selected file and line amount? What module or functionality induced it? These questions can information your investigation and point you toward the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can substantially speed up your debugging approach.
Some errors are vague or generic, As well as in Those people instances, it’s important to look at the context by which the error transpired. Check associated log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede bigger troubles and supply hints about potential bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, supporting you pinpoint difficulties faster, reduce debugging time, and become a a lot more successful and self-confident developer.
Use Logging Sensibly
Logging is one of the most potent resources in the developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through progress, Data for basic occasions (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true difficulties, and FATAL in the event the process can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and slow down your procedure. Target crucial events, condition adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping through code isn’t attainable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging technique, you could reduce the time it requires to identify issues, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This way of thinking allows break down complicated concerns into manageable areas and observe clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much suitable facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations not too long ago been created to your codebase? Has this challenge happened in advance of underneath related conditions? The objective would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in a very controlled environment. For those who suspect a particular perform or ingredient, isolate it and confirm if the issue persists. Like a detective conducting interviews, check with your code queries and let the effects direct you nearer to the truth.
Pay near interest to compact specifics. Bugs often cover within the the very least anticipated sites—like a lacking semicolon, an off-by-a single mistake, or maybe a race issue. Be comprehensive and patient, resisting the urge to patch The problem with out absolutely comprehension it. Temporary fixes may perhaps conceal the actual issue, just for it to resurface later.
And finally, maintain notes on Anything you experimented with and learned. Just as detectives log their investigations, documenting your debugging procedure can help you save time for potential challenges and aid Many others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical competencies, strategy complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated units.
Create Assessments
Crafting tests is one of the most effective strategies to here help your debugging skills and General growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when generating improvements towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These compact, isolated checks can immediately expose no matter if a selected bit of logic is Doing work as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Next, combine integration exams and finish-to-end checks into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re specifically useful for catching bugs that manifest in intricate methods with multiple parts or solutions interacting. If something breaks, your checks can let you know which part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a element correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—helping you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at trouble-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the qualifications.
Breaks also support avoid burnout, especially all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to move all-around, stretch, or do a little something unrelated to code. It could feel counterintuitive, In particular under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or comprehending and assist you to Develop stronger coding routines shifting forward.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a short produce-up, or a quick understanding-sharing session, encouraging Some others avoid the same challenge boosts group performance and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In any case, a lot of the ideal builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — even so the payoff is large. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.